728x90
이벤트와 효과
제이쿼리를 이용하면 웹 페이지에서 발생되는 이벤트를 쉽게 처리할 수 있다.
마우스, 폼 , 윈도우 등의 조작으로 인해 발생되는 이벤트를 제이쿼리로 처리하고, 또 페이지 요소들에 대해 숨기기(hide), 페이드, 슬라이드(slide), 그리고 애니메이션(animation) 등 다양한 효과를 주는 제이쿼리 메써드도 알아본다.
이벤트는 웹 페이지에서 사용자의 마우스나 키보드 조작에 의해 발생되는 사건/일/작업/행동을 말한다.
예를 들어 사용자가 키보드를 클릭하면 클릭 이벤트가 발생한다.
제이쿼리를 이용하면 웹 페이지에서 발생하는 이벤트를 쉽게 처리 할 수 있다. 발생된 이벤트를 처리해 주는 함수를 이벤트 메써드라고 한다.
제이쿼리에서는 마우스이벤트, 키보드 이벤트, 문서 이벤트, 브라우저 이벤트 등이 발생했을 때 이를 처리해주는 다양한 메써드가 존재한다.
마우스 이벤트 | click ( ) | 선택 요소의 클릭 |
dblclick ( ) | 선택 요소의 더블클릭 | |
mouseenter ( ) | 선택 요소에서 마우스 포인터가 들어갔을 때 | |
mouseleave ( ) | 선택 요소에서 마우스 포인터가 벗어났을 때 |
더보기
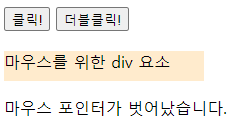
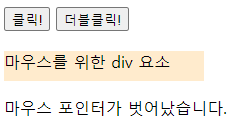
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<script>
$(document).ready(function(){
$("#btn1").click(function(){ //event handler
$("#show").text("클릭했습니다");
});
$("#btn2").dblclick(function(){ //event handler
$("#show").text("더블클릭했습니다");
});
$("#box").mouseenter(function(){ //event handler
$("#show").text("마우스 포인터가 들어왔습니다.");
});
$("#box").mouseleave(function(){ //event handler
$("#show").text("마우스 포인터가 벗어났습니다.");
});
});
</script>
<style>
#box { width: 200px; height: 30px; margin-top: 20px; background-color: blanchedalmond;}
</style>
<title></title>
</head>
<body>
<button id="btn1">클릭!</button>
<button id="btn2">더블클릭!</button>
<div id="box">마우스를 위한 div 요소</div>
<p id="show"></p>
<!-- 위에서 show 지정자가 text() 메세지를 보이므로 p로 처리 -->
</body>
</html>
키보드 이벤트 | keypress ( ) | 키보드가 눌려졌을 때 |
keydown ( ) | 키보드가 눌려지고 있을 동안 | |
keyup ( ) | 키보드가 눌려졌다가 뗄 때 |
더보기
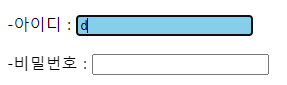
입력중...
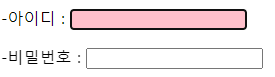
입력 받다가 뗄 때
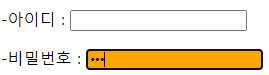
눌러져 있는 동안
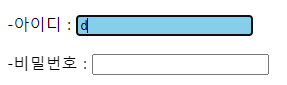
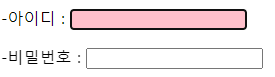
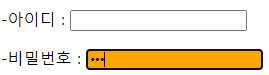
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<script>
$(document).ready(function(){
$("p:eq(0) input").keydown(function(){
$(this).css("background-color","skyblue") // this는 위에서 선택한 p:eq에 첫번째
});
$("p:eq(0) input").keyup(function(){
$(this).css("background-color","pink")
});
$("p:eq(1) input").keypress(function(){
$(this).css("background-color","orange")
});
});
</script>
<style>
#box { width: 200px; height: 30px; margin-top: 20px; background-color: blanchedalmond;}
</style>
<title></title>
</head>
<body>
<P>-아이디 : <input type="text"></P>
<p>-비밀번호 : <input type="password"></p>
</body>
</html>
폼이벤트 | focus ( ) | <input type="text">등 요소에 마우스 포커스가 될 때 |
blur ( ) | <input type="text">등 요소에 | |
change ( ) | <input>, <select> 등 요소에서 값이 변경되었을 때 |
더보기
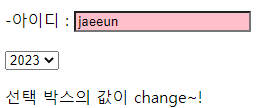
select 값을 바꾸고 마우스 커서 옮김
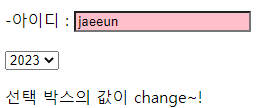
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<script>
$(document).ready(function(){
$("input").focus(function(){
$(this).css("background-color","yellow");
});
$("input").blur(function(){
$(this).css("background-color","pink");
});
$("select").change(function(){
$(show).text("선택 박스의 값이 change~!");
});
});
</script>
<style></style>
<title></title>
</head>
<body>
<P>-아이디 : <input type="text"></P>
<select>
<option>2020</option>
<option>2021</option>
<option>2022</option>
<option>2023</option>
<option>2024</option>
</select>
<p id="show"></p>
</body>
</html>
문서/윈도우 이벤트 | ready ( ) | 문서 객체 모델의 요소들이 모두 로드 되었을 때 발생되었을 때 |
resize ( ) | 브라우저 창의 크기가 변경되었을 때 | |
scroll ( ) | 스크롤 바를 움직였을 때 |
더보기


브라우저 크기를 조절 할 때마다 측정
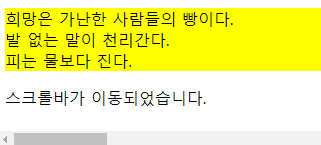


<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<script>
$(document).ready(function(){
$(window).resize(function(){
$("span:eq(0)").text(window.innerWidth);
$("span:eq(1)").text(window.innerHeight);
});
});
</script>
<style></style>
<title></title>
</head>
<body>
<P>브라우저 창 크기 :<span></span>  <span></span></P>
</body>
</html>
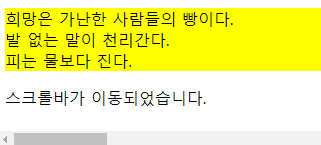
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<script>
$(document).ready(function(){
$(window).scroll(function(){
$("#show").text("스크롤바가 이동되었습니다.");
});
});
</script>
<style></style>
<title></title>
</head>
<body>
<div style="width: 2500px; overflow:auto; background-color:yellow;">
희망은 가난한 사람들의 빵이다. <br>
발 없는 말이 천리간다.<br>
피는 물보다 진다.
</div>
<p id="show"></p>
</body>
</html>
이벤트 등록 " ON ( ) "
앞에서는 하나의 이벤트가 발생했을 때 그에 해당되는 이벤트 메써드로 발생된 이벤트를 처리했다.
예를 들어 사용자가 마우스를 클릭하면 click 이벤트가 발생되고 click 메써드로 처리하고,
mouseenter 이벤트가 발생되면 이 이벤트를 처리하는 mouseenter() 메써드로 처리 할 수 있다.
제이쿼리에서는 on( ) 매써드를 사용해서 발생되는 이벤트를 이벤트 핸들러에 직접 등록할 수 있다.
" on( ) " 메써드 기본 문법
▪ 하나의 이벤트 등록 on() 매써드
$(선택자).on(“이벤트”, funcrion() {
이벤트_핸들러_코드1;
}); 구문이다.
▪ 다수의 이벤트 등록 on() 메써드
$(선택자).on( {
이벤트1 : function() {
이벤트_핸들러_코드1;
},
이벤트2 : function() {
이벤트_핸들러_코드2;
},
이벤트3 : function() {
이벤트_핸들러_코드3;
},
...
}
}) 구문이다.
더보기

마우스가 박스 안에 있을 때

마우스를 밖에다 놓았을 때

클릭 했을 때

더블 클릭 했을 때




<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<script>
$(document).ready(function(){
$("p").on( {
mouseenter : function() {
$(this).css("background-color","yellow");
},
mouseleave : function() {
$(this).css("background-color","pink");
},
click : function() {
$(this).css("background-color","skyblue");
},
dblclick : function() {
$(this).css("background-color","red");
}
});
});
</script>
<style></style>
</head>
<body>
<p style="border : solid 2px black;">발 없는 말이 천리간다.</p>
</body>
</html>
'일단 해보는 코딩 > jQuery' 카테고리의 다른 글
[jQuery] 제이쿼리 위젯 (2) | 2022.07.14 |
---|---|
[jQuery] jQuery효과, 애니메이션 효과 (0) | 2022.07.14 |
[jQuery] 요소 탐색 (0) | 2022.07.12 |
[jQuery] 선택자 (0) | 2022.07.12 |
[jQuery] jQuery의 기초 (0) | 2022.07.11 |