728x90
다형성(polymorphism) 이란?
한 가지의 타입이 여러 가지 형태의 인스턴스를 가질 수 있는 것
부모-자식 상속 관계에 있는 클래스에서 상위 클래스가 동일한 메시지로 하위 클래스들을 서로 다르게 동작시키는 객체 지향 원리이다. 다형성을 활용하면 부모 클래스가 자식 클래스의 동작 방식을 알수 없어도 오버라이딩을 통해 자식 클래스를 접근가능하다.
다형성의 여러 방법 : 부모 자식간의 casting(형 변환)
1. 업 캐스팅(upcasting) : 자식 클래스의 객체가 부모클래스의 참조 변수로 형 변환 되는 것
구조 : 부모클래스 변수 = 자식 객체값;
더보기
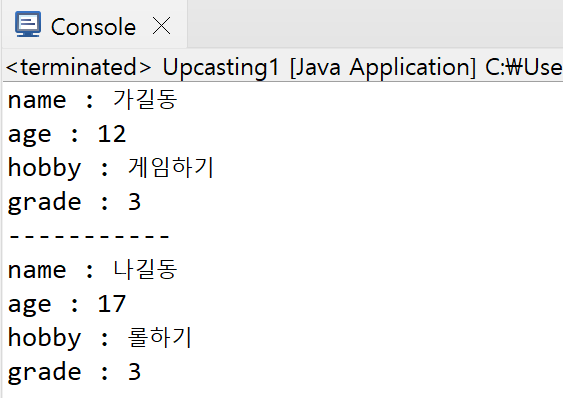
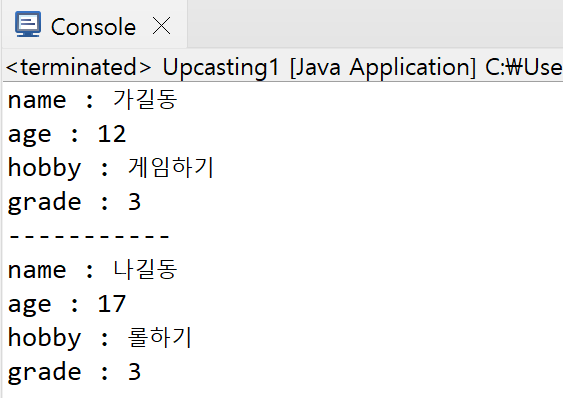
package Home25;
public class Upcasting1 {
public static void main(String[] args) {
// TODO Auto-generated method stub
Student st1 = new Student("가길동",12,"게임하기",3);
st1.info();
System.out.println("-----------");
Human h1 = new Student("나길동",17,"롤하기",3);
h1.info();
//h1.study();
//System.out.println(h1.grade);
// Upcasting이 되었기 떄문에 Human 클래스의 객체 변수는 grade변수 사용 불가, Human 클래스의 객체 변수는 study()메서드 호출 불가!
}
}
class Human {
String name;
int age;
String hobby;
public Human(String name, int age, String hobby) {
this.age = age;
this.hobby = hobby;
this.name = name;
}
void info() {
System.out.println("name : " + name);
System.out.println("age : " + age);
System.out.println("hobby : " + hobby);
}
}
class Student extends Human{
int grade;
public Student(String name, int age, String hobby, int grade) {
super(name,age,hobby);
this.grade=grade;
}
void info() { // 오버라이딩
super.info();
System.out.println("grade : " + grade);
}
void study() {
System.out.println("공부하기");
}
}
2. 다운 캐스팅(downcasting) :
구조 :
예제모음
더보기
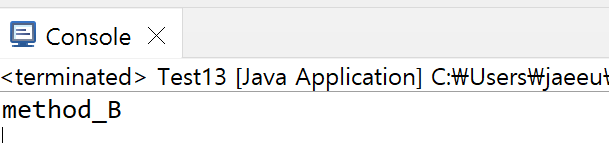
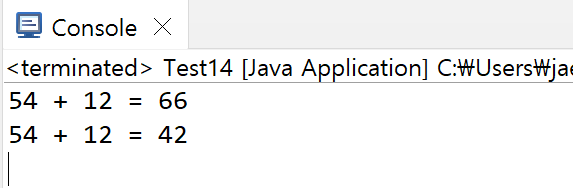
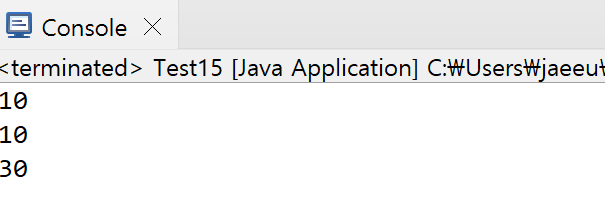
예제 1)
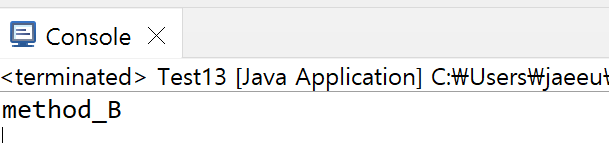
package Java13;
class A {
void methodA() {
System.out.println("method_A");
}
}
class B extends A {
void methodA() { // method() 오버라이딩
System.out.println("method_B");
}
void methodC() {
System.out.println("method_C");
}
}
public class Test13 {
public static void main(String[] args) {
A a=new B(); // 다형성
a.methodA();
// a.methodC(); # 오류!! Class C에서 methodA()를 오버라이딩하지 않았다.
}
}
예제 2)
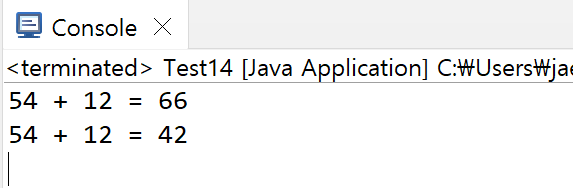
package Java13;
abstract class Calc2 { // 추상 클래스
int a ;
int b ;
abstract int result() ;
void printResult() {
System.out.println(result()) ;
}
void setData(int m, int n) {
a = m ;
b = n ;
}
}
class Plus extends Calc2 {
int result() { // result() 메서드 오버라이딩, 다형성
return a + b ;
}
}
class Minus extends Calc2 {
int result() {
return a - b ;
}
}
class Test14 {
public static void main(String [] args) {
int x = 54, y = 12 ;
Calc2 calc1 = new Plus() ;
Calc2 calc2 = new Minus() ;
calc1.setData(x, y) ;
calc2.setData(x, y) ;
System.out.print(x + " + " + y + " = ") ;
calc1.printResult( ) ;
System.out.print(x + " + " + y + " = ") ;
calc2.printResult( ) ;
}
}
예제 3)
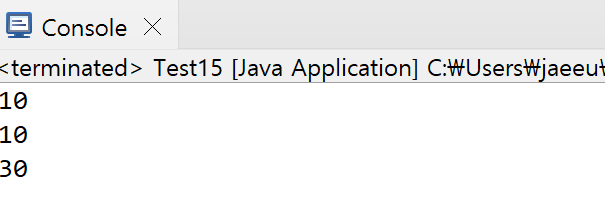
package Java13;
class AB {
int methodA(int a, int b) {
return a+b;
}
}
class BC extends AB {
int methodA(int a, int b) {
return a-b;
}
void methodBC() {
System.out.println("hi");
}
}
public class Test15 {
public static void main(String[] args) {
int a, b;
a= 20;
b= 10;
AB obj1 = new BC(); // 다형성
System.out.println(obj1.methodA(a,b));
BC obj2 = new BC();
System.out.println(obj2.methodA(a,b));
AB obj3 = new AB();
System.out.println(obj3.methodA(a,b));
}
}
'일단 해보는 코딩 > Java' 카테고리의 다른 글
[Java] Method(메서드) 정리 (0) | 2022.08.05 |
---|---|
[Java] IO(Input/Output) - 입출력 (0) | 2022.08.03 |
[Java] Ramda 식 (0) | 2022.08.03 |
[Java] Wrapper (boxing/unboxing) (0) | 2022.08.03 |
[Java] 컬렉션 프레임윅(Collections Framework) - ArrayList (0) | 2022.08.03 |